Python
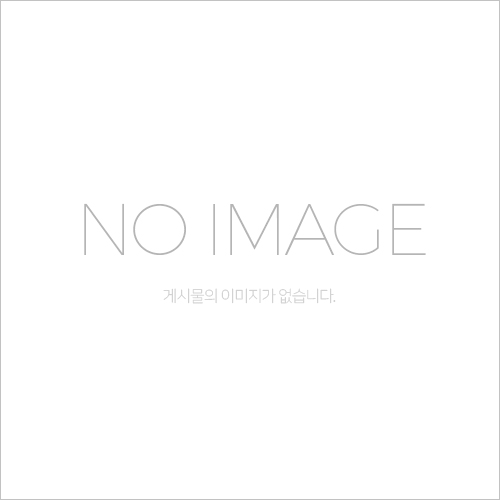
12345678910111213141516171819202122232425262728293031323334353637383940414243444546from http.client import HTTPSConnectionfrom bs4 import BeautifulSoup # Webcrawlling# HTML Parsing # JSON / XML # DB에 있는 데이터를 남들이 보기 편하게 표현해 놓은 것 # HTML# 웹사이트 이쁘게 보이도록 - 디자인 # 소스보기 (view-source:)# view-source:https://m.daum.net/ hc = HTTPSConnection("m.daum.net")hc.request("GET","/")resBody = hc.getresponse().read(..
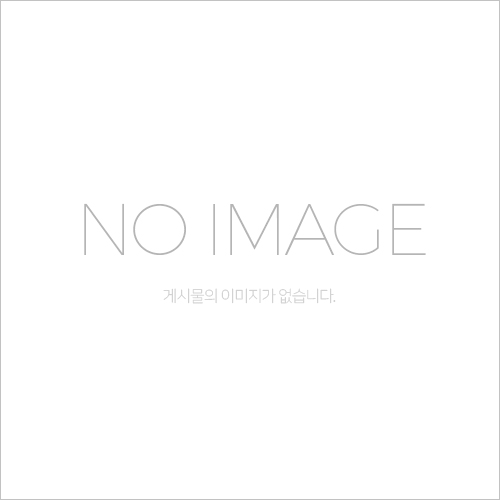
1234567891011121314151617181920212223242526272829303132from urllib.parse import quotefrom http.client import HTTPSConnectionfrom json import loads # # JSON Parsing# https://developers.kakao.com/docs/latest/ko/daum-search/common # l = [1, 2, 3] # Python : List , JS : array# d = {"height" : 180, "weight" : 80} # Python : dict , JS : object # 검색어 입력받기, 한글 처리q = quote(input("뭐 : ")) # 요청헤더 설정h = {"A..
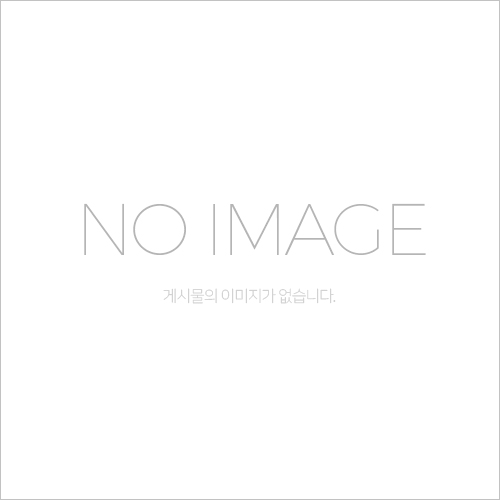
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051from http.client import HTTPSConnectionfrom urllib.parse import quotefrom xml.etree.ElementTree import fromstring # DEV.naver.com# X-Naver-Client-Id : ID# X-Naver-Client-Secret : PW # https://developers.naver.com/products/search/ # 요청 주소 : https://openapi.naver.com/v1/search/shop.xml# query# X-Naver-Clie..
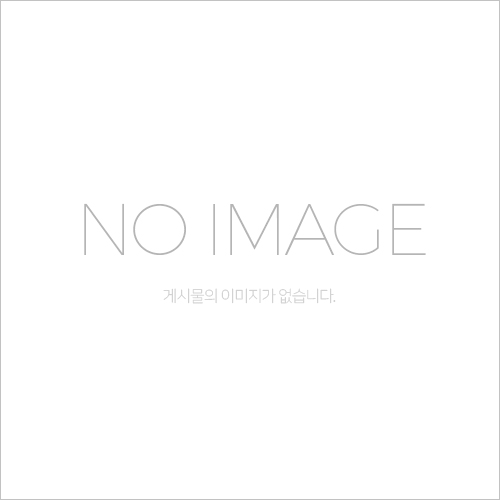
123456789101112131415161718192021222324252627282930313233343536373839404142434445# HTTP 통신# HTTPCOnnection과 HTTPSConnection 구분 # HTTPConnection("서버주소")# HTTPConnection("www.kma.go.kr") # hc.request("요청방식", "남은주소") 파라메터까지 전부# hc.request("GET", "/wid/queryDFSRSS.jsp?zone=1132052200") /빼먹지 말기 # 응답# res = hc.getresponse() # 응답 받은 내용# resBody = res.read() # 한글 처리해서 출력# resBody.decode() # XML parsing#..
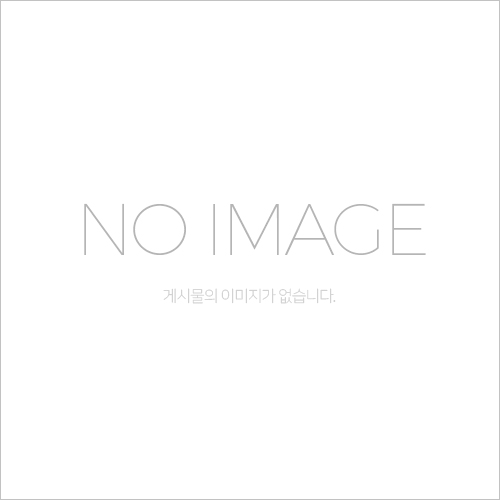
12345678910111213141516171819202122232425262728293031323334353637383940414243444546474849505152535455565758596061# Java Exception# 언제 하는지 몰라도 상관 없음# 하기 싫은데 무조건 해야함# 직접 처리 : try - catch - finally# 미루기 : throws # error : 컴파일할때(소스를 기계어로 바꿀때) 실패하는 것# warning : 정리안된 소스# exception: 실행 중 예외적인 상황이 발생해서 정상적으로 안되는 # Python Exception# 하기싫으면 안해도 됨# 직접 처리 : try - except - else - finally# 인터프리터 방식 - exception..
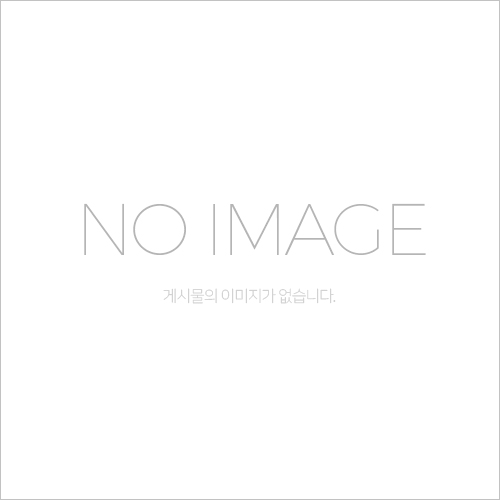
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566# 다중상속# Java - 공식적으로 불가능(interface로 흉내만 냄)# Python - 가능 # Python# 생성자가 상속됨# 다중 상속 가능# overloading 안됨# 이름 중복 시 -> 먼저 받은걸로 선택 class Avengers: def __init__(self, realName): self.realName = realName def attack(self): print("공격") def printInfo(self): print(self.realName) class Hu..
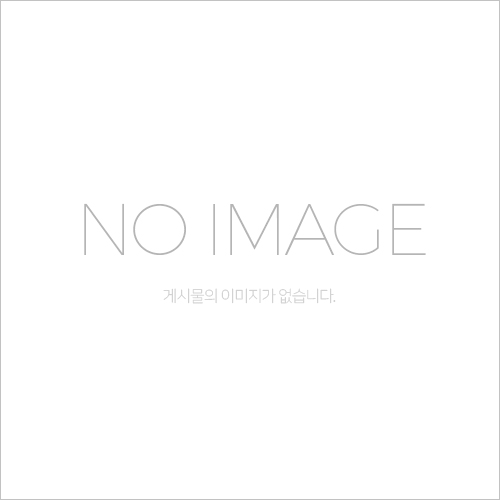
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748# Java# 생성자는 상속이 안됨 # Python# 생성자 상속이 됨# 멤버변수를 생성자에서 결정하니 # 생성자를 상속시켜주지 않으면 -> 멤버변수가 상속되지 않음 class Avengers: def __init__(self, name, age): print("어벤져스 등장") self.name = name self.age = age def attack(self): print("공격") def printInfo(self): print(self.name) print(self.age) # Avengers를 상속받는 IronManclass IronMan(..
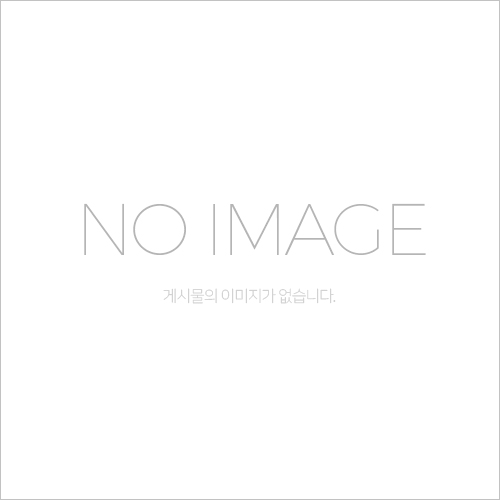
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 class Cat: def __init__(self, name, age): self.name = name self.age = age def printInfo(self): print(self.name) print(self.age) if __name__ == "__main__": from animal.wild import Tiger t = Tiger(10) t.showInfo() cs 먼저 animal이라는 package에 pet이라는 모듈에 Cat 클래스가 있다. 1 2 3 4 5 6 7 8 9 10 11 12 13 # 다른 개발자가 만든 고양이 # from animal.pet import Cat import animal.pet as ap #..
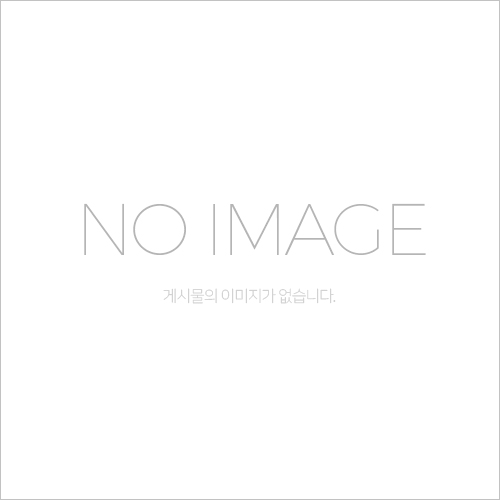
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253545556575859# Java# 자기가 작업한거 공유하는 문화# 클래스명 중복이 생길 수 있다.# 클래스명이 중복될 때 구분할 수단 : package# package명은 전세계적으로 중복 되지 않아야한다.# 모든 class는 package에 소속되어 있어야한다.# 패키지명.클래스로 사용# 같은 패키지 or java.lang 소속 or import 하면 패키지명 생략 가능하다 # Python# 자기가 작업한거 공유하는 문화# 클래스명 중복이 생길 수 있다.# 클래스명이 중복될 때 구분할 수단 : package# package명 규칙..